Apache Spark Performance: 5 Must-Used Optimization Techniques
Big Data
5 MIN READ
March 29, 2025
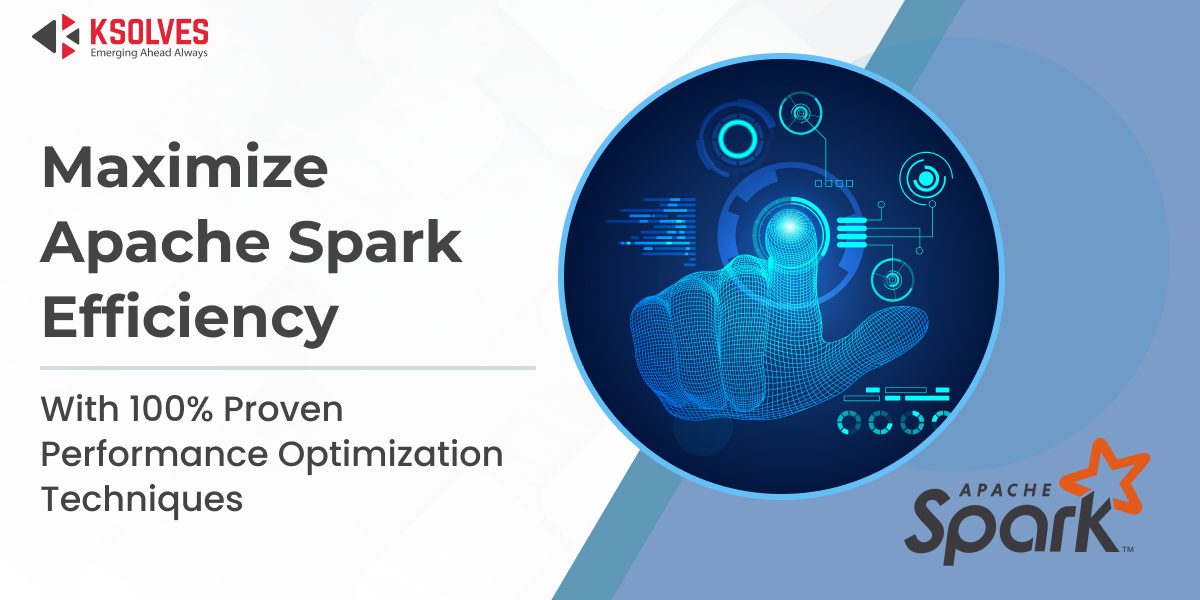
Data has become the driving force behind business success in today’s fast-paced digital world. It fuels decision-making, uncovers new opportunities, and powers innovation. However, as businesses generate and collect massive volumes of data, managing, analyzing, and extracting meaningful insights from it becomes a daunting challenge. That’s where big data analysis comes to the rescue. It enables you to interpret information from complex data sets that would be difficult using standard methods. Large-scale data analysis improves decision-making and the entire consumer experience. It also optimizes resources and reduces waste through smart allocation. Businesses require potent tools like Apache Spark to fully realize big data’s potential.
This open-source data processing platform speeds up large-scale data processing. Its sturdy architecture speeds the process while maintaining the accuracy of the output. But as they say, “ All that glitters is not gold.” Similarly, Apache Spark has complexities and bottlenecks, which can affect its performance. The inefficient configurations slow down the job and clog your resources. That’s why optimizing Apache Spark becomes crucial. In this article, we will explore the top essential Apache Spark performance optimization techniques for driving better results.
Understanding the Spark Ecosystem
Master-Worker Model
Apache Spark uses a master-slave architecture containing the following key components: a driver program, cluster managers, and executor and worker nodes. The driver program acts as the control center, responsible for job planning and execution. It converts the code into a directed acyclic graph that helps Spark understand the workflow. The driver sends the task to the other workers or executors and monitors their progress.
The cluster manager is the one who manages the hardware installed in the system. Performance tuning in Apache Spark ensures the efficient utilization of the cluster manager’s resources for faster processing. There are different types of cluster managers available in Spark, each having its functionalities. Next, comes the executors who perform work in the Spark eco-system. They receive the task, execute it, and then send the feedback to the driver. So, the complete Spark architecture is designed to provide flexibility and fault tolerance.
Spark Abstractions
Abstractions are high-level programming interfaces that simplify working with large data sets. They simplify data work by abstracting the complexities of distributed systems, making it easier for developers to use the Apache Spark tuning guide to scale applications. RDDs are the most basic abstractions in Spark and can be processed in parallel across the cluster of machines. Data frames, which are the higher-level abstractions, are optimized for structured data.
Data sets are extensions of data frames and combine the benefits of RDDs and data frames. They provide type safety, allowing the compiler to check the data type at the time of compiling, eliminating the chances of run-time errors. Apache Spark also has Spark SQL abstraction, which allows users to run SQL queries.
Optimization Techniques To Supercharge Apache Spark
1. Use Dataframes/Datasets over RDDs
Today, datasets and data frames are preferred over RDDs because they are connected to optimizing tools like Catalyst and Tungsten. This makes them more efficient and faster in operations. Dataframes are like tables in a database that organize data into rows and columns, which makes it convenient for the user to work with them. On the other hand, data sets are like data frames with extra safety.
Code:
val df = spark.read.json(“examples/src/main/resources/people.json”)
case class Person(name: String, age: Long)
// Encoders are created for case classes
val caseClassDS = Seq(Person(“Andy”, 32)).toDS()
// Encoders for most common types are automatically provided by importing spark.implicits._
val primitiveDS = Seq(1, 2, 3).toDS()
// Performing a transformation on Dataset
primitiveDS.map(_ + 1).collect() // Returns: Array(2, 3, 4)
// DataFrames can be converted to a Dataset by providing a class. Mapping will be done by name
val path = “examples/src/main/resources/people.json”
val peopleDS = spark.read.json(path).as[Person]
2. Data Serialization
Serialization allows easy storage and transmission of the object across the distributed cluster. In Apache Spark Performance Optimization, serialization is crucial for efficiently transmitting data between different cluster nodes and compactly storing data in memory. It also helps reduce memory footprints, which is crucial for Spark as it often deals with massive data. Thus, serializing data minimizes memory consumption, reducing the likelihood of out-of-memory errors.
To implement this in Spark, you can configure KryoSerializer for efficient serialization, as shown in the following code:
conf.set(“spark.serializer”, “org.apache.spark.serializer.KryoSerializer”)
Code:
val conf = new SparkConf().setMaster(…).setAppName(…)
conf.registerKryoClasses(Array(classOf[MyClass1], classOf[MyClass2]))
val sc = new SparkContext(conf)
3. Caching and Persistence
These are powerful techniques for optimizing Spark performance. Caching involves storing the intermediate computation results for subsequent use. This eliminates the expensive recalculation of those operations every time. Thus, caching and persistence boost speed and help in efficient resource utilization. Spark provides different storage levels to control how data is persisted, which is crucial for performance tuning in Apache Spark.
How to Cache and Persist Data?
- For caching:
df.cache()
# or
df.persist()
- For persistence with a specific storage level:
from pyspark import StorageLevel
df.persist(StorageLevel.MEMORY_AND_DISK)
4. Data Partitioning
It means dividing the extensive data set into smaller and more manageable portions. This practice allows Spark to process the data simultaneously across different machines to speed up the process. It is technically known as parallel processing. Data partitioning also ensures load balance by allocating work across all machines in the cluster. This makes the entire system more efficient. The thumb rule for good partitioning is to have 2-3 tasks for each CPU.
- Checking the Number of partitions:
num_partitions = df.rdd.getNumPartitions()
print(f”Number of partitions: {num_partitions}”)
- Checking the size of each partition:
partition_sizes = df.rdd.mapPartitions(lambda x: [sum(1 for _ in x)]).collect()
print(“Size of each partition:”)
for i, size in enumerate(partition_sizes):
print(f”Partition {i}: {size}”)
5. Shuffle Operations
These operations can be necessary for some tasks, but they can also slow down the processing when not handled properly. Therefore, streamlining Shuffle operations is one of the best practices for Spark optimization. Spark performs souffle operations when it needs to reorganize the data into a group based on some keys. Early filtration and removing unnecessary data can effectively minimize the impact of shuffling. Other methods include broadcast joins, data partitioning, and map-side reductions.
- Inefficient Example (Late Filtering):
df_bad = df.groupBy(“department”).agg(F.avg(“salary”).alias(“avg_salary”)).filter(F.col(“department”) != “IT”)
- Efficient Example (Early Filtering):
df_good = df.filter(F.col(“department”) != “IT”).groupBy(“department”).agg(F.avg(“salary”).alias(“avg_salary”))
6. User-Defined Functions (UDFs)
A Spark user-defined function is a custom function that a user can create to perform a specific task. It allows them to add custom logic for performing complex operations or use external libraries. To make things more efficient, first, check whether Spark already has a built-in function or not. If yes, then use that in-built function because it will always be faster than UDFs. Next, use Panda UDFs, as they process data in batches rather than in rows. This Apache Spark Performance Optimization technique improves the speed.
Here’s an example comparing a UDF to a built-in function:
from pyspark.sql.functions import udf, col, upper
# Using a UDF
upper_udf = udf(lambda x: x.upper())
df_udf = df.withColumn(“upper_name”, upper_udf(col(“name”)))
# Using a built-in function
df_builtin = df.withColumn(“upper_name”, upper(col(“name”)))
7. Data Skew
It happens when some values or keys are more common than others within data. This creates a non-balanced workload, so some tasks may take longer to finish. In Spark, there are several methods through which you can fix data skew. For example, the salting technique helps spread the unevenly distributed data more evenly across partitions. Spark 3.0 introduces AQE, which is particularly beneficial for handling data skews. It automatically detects and optimizes skew joins by splitting them into smaller, more distributed partitions. It also keeps an eye on data distribution while running a query, and in case of any problem encountered, it changes the plan or uses a better strategy.
Recently, Apache released its latest version, Apache Spark 3.5.3, which offers several enhancements, such as Spark Connect for providing remote connectivity to the Spark cluster, enhanced query optimization, a new algorithm for better machine learning, simplified API, and improved SQL query performance.
Other Ways for Optimizing Apache Spark Performance
Broadcast Joins Optimization → When working with large datasets, traditional join operations can be resource-intensive, leading to slow query performance. Spark’s broadcast join optimization helps tackle this issue by broadcasting smaller tables across worker nodes, reducing data shuffling and improving processing speed. This technique is particularly effective when joining a small dataset with a much larger one, ensuring faster execution and reduced memory consumption. Using the broadcast() function in Spark efficiently handles such scenarios, making queries more responsive and cost-effective.
Cost-Based Optimizer (CBO) → Apache Spark’s Cost-Based Optimizer (CBO) enhances query performance by choosing the most efficient execution plan based on statistics about data distribution and size. Instead of relying on fixed rule-based optimization, CBO analyzes the cost of different query plans and selects the most optimal one. This approach reduces computational overhead and improves overall efficiency. Enabling CBO in Spark SQL ensures better join strategies, optimized aggregation functions, and reduced data movement, leading to faster and more reliable query execution.
Dynamic Partition Pruning → When dealing with partitioned tables, Spark can struggle with unnecessary data scans, leading to slow performance. Dynamic Partition Pruning (DPP) addresses this issue by dynamically filtering partitions at runtime based on query conditions. This means that only relevant partitions are scanned, reducing I/O overhead and improving execution speed. DPP is especially useful in scenarios where join queries involve partitioned tables, making Spark more adaptive to runtime data constraints and significantly enhancing query performance.
By leveraging broadcast joins, cost-based optimization, and dynamic partition pruning, businesses can maximize Apache Spark’s efficiency and scalability. These techniques ensure faster query execution, reduce resource consumption and enhance overall big data processing capabilities. Adopting these optimizations empowers organizations to handle vast datasets seamlessly, enabling data-driven decisions with speed and precision.
Monitoring and Configuration Tuning
Monitoring the Spark application is essential because it helps users identify performance bottlenecks, making it a key aspect of any Apache Spark tuning guide. They can then work on these problems to improve the application’s performance. Sparks comes with various tools that can help users in this process. Spark UI is a tool that showcases your job’s key performance metrics. Here’s how you can use Spark UI to improve your performance.
- Identify long-running jobs
- Detect excessive data shuffling
- Monitor memory users
- Track garbage collections
Other Spark Monitoring Tools Beyond Spark UI
Apart from inbuilt tools, third-party tools are available in Apache Spark to enhance visibility and insights. These tools can recommend changes for better utilization of resources. A few essential third-party tools are:
Ganglia
It is a distributed and highly scalable cluster monitoring tool that allows monitoring of memory CPU and other metrics, which is crucial for tracking cluster health. Three main packages simplify the monitoring process: Gmond, Ganglia Web, and Gmetad.
YARN Resource Manager
It is an Apache Spark Performance Optimization tool that manages and allocates resources across different applications. It helps track available resources on each node in the cluster and then allocate them based on scheduling policies.
Datadog
Offers several benefits due to its robust features and seamless integration capabilities. It provides centralized monitoring to understand better how Spark interacts with the rest of the system.
Best Practices for Spark Optimization Monitoring and Tuning
Set a Baseline
The first thing that you must do is to understand how your application is performing under normal conditions. This will help you identify something unusual that slows down your system.
Make Gradual Changes
One key Apache Spark performance optimization technique is avoiding making multiple changes at once, as it can complicate root cause analysis. Each change you make has a different impact on the system. Therefore, the best way to adjust the setting little by little is by watching how it impacts the performance.
Regularly Reviewing Your Spark Settings
Regular monitoring can help you maintain the optimal performance of your Spark applications. It can also help you adjust your settings to ensure they align with the new data as Spark updates and new features may be introduced.
Outcome
Optimizing your Spark is an ongoing process that can help you overcome performance bottlenecks for efficient data processing. It also enables Spark to run faster, use resources more effectively, and provide better data insights. By using these Apache Spark performance optimization techniques, you can unlock its full potential. Ksolves is a trusted name for Apache Spark, consisting of certified professionals who bring vast knowledge. Our end-to-end services ensure that we cover everything from strategy to implementation for the success of your project. We use smart analytics to empower your businesses with valuable insights that drive growth. At Ksolves, we offer scalable, cost-effective Apache Spark development services to optimize your big data projects for performance.
So, partner with us and leverage our experience to drive sustainable growth to your business!
AUTHOR
Big Data
Anil Kushwaha, Technology Head at Ksolves, is an expert in Big Data and AI/ML. With over 11 years at Ksolves, he has been pivotal in driving innovative, high-volume data solutions with technologies like Nifi, Cassandra, Spark, Hadoop, etc. Passionate about advancing tech, he ensures smooth data warehousing for client success through tailored, cutting-edge strategies.
Share with